For every Laravel project I do, probably one of the first things as part of giving a custom look and feel to the laravel project that I do is to customize the error pages of the website in Laravel. So, this post is going to be a quick guide about how to create a custom 404 page in Laravel 7.x.
The 404 error on a Laravel website is an exception that is checked and handled by Laravel in the app/Exceptions/Handler.php class. In fact, every exception generated by the Laravel website can be configured in this file and responded to.
How to create a custom 404 page in Laravel?
This guide on returning a custom 404 response for a Laravel web application usess Laravel 7.9.2.
The default Laravel 404 page that gets returned is very pale and simple. Let’s see how to return a custom 404 page in our Laravel project.
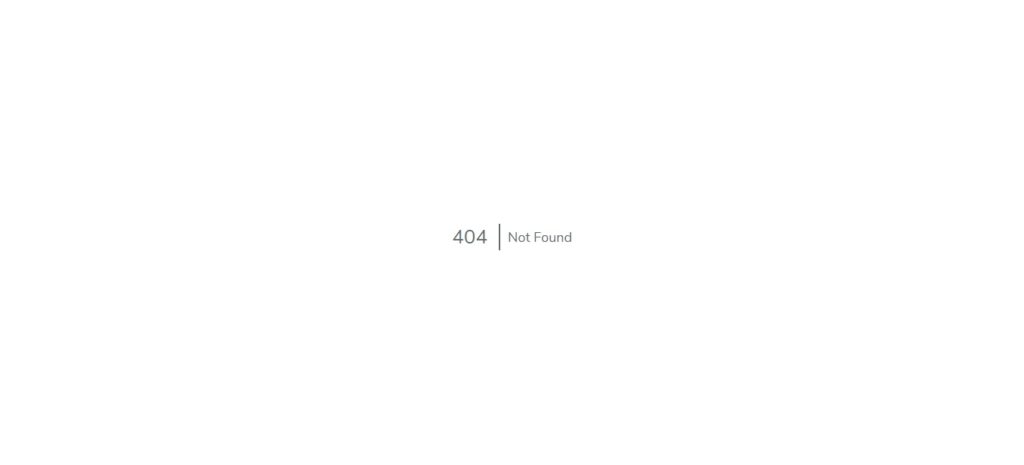
Create and set up a new Laravel project
This tutorial on setting up and returning a custom 404 page in Laravel project assumes that you already have your Laravel web application set-up and running. If you do not know how to create a new Laravel project, it is fairly simple.
All you have to do is run the following command and you will have a new Laravel project.
laravel new project-name
composer require laravel/ui
Modifying the Handler.php file
As already mentioned above, all the magic regarding exception handling in Laravel happens in the app/Exceptions/Handler.php file. So, let’s go and do the necessary changes there.
To show a custom not found page in Laravel, we need to modify the ‘render‘ method in this file. The change that needs to be done will look as follows:
public function render($request, Throwable $exception)
{
if ($this->isHttpException($exception)) {
if ($exception->getStatusCode() == 404) {
return response()->view('errors.' . '404', [], 404); //errors is a folder inside views
}
}
return parent::render($request, $exception);
}
NOTE: If getStatusCode throws an error (depending on your Laravel version), use the following code instead:
public function render($request, Throwable $exception)
{
if ($this->isHttpException($exception)) {
if ($exception->getCode() == 404) {
return response()->view('errors.' . '404', [], 404); //errors is a folder inside views
}
}
return parent::render($request, $exception);
}
And that should be it.
Dissecting the code
So, what we basically did in the code that we added in the render method to make it redirect a custom 404 error page can be summarised in the following points.
- We check if the exception is an HttpException.
- If it is indeed a HTTP exception, we check if it is a 404 error by checking the exception’s StatusCode.
- If the StatusCode is indeed 404, we return the response with a view called 404.
- Please note that you must create a view file named ‘404.blade.php’.
You can choose to give a different name to your view file. If you do so, do not forget to change the same in the line above where you return the response.
In my case, my custom 404 file is called ‘404.blade.php‘ and resides in a folder called ‘errors‘, under the ‘resources\views‘ directory.
Bonus tip – Custom Pages for other HttExceptions in Laravel.
If you notice the code above, you must have already noticed that we can check for other HTTPS exceptions like 500, etc.
All you have to do is check the StatusCode and then return a relevant view accordingly.
Finally, this is how my custom 404 page in Laravel looked.
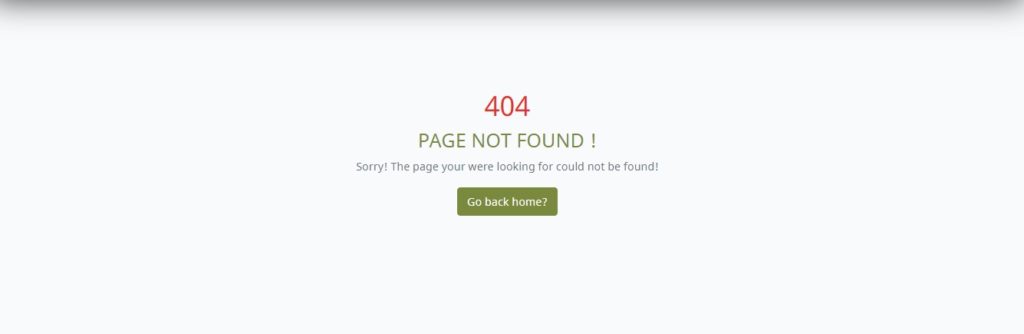
That’s it.
2 thoughts on “How to create a custom 404 page in Laravel?”
Pingback: How To Fix Laravel 7.x Specified key was too long error?
Pingback: What do I do if My 2FA token is always invalid? - Rajiv Verma